In Iterate Map in JavaScript, you can iterate over the keys and values of an object or map using a for...of
loop or a forEach
method.
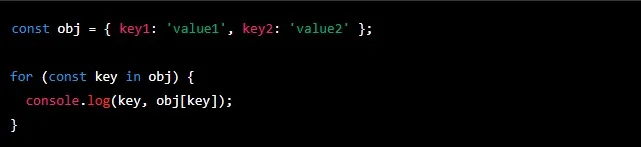
Table of Contents
iterate map in javascript Example.
Here’s an example of using a for...of
loop to iterate over the keys and values of a map:
const map = new Map([
['key1', 'value1'],
['key2', 'value2']
]);
for (const [key, value] of map) {
console.log(key, value);
}
This will output:
key1 value1
key2 value2
You can also use the forEach
method to iterate over the keys and values of a map:
map.forEach((value, key) => {
console.log(key, value);
});
This will have the same output as the for...of
loop above.
Keep in mind that a Map
is a specific data structure in JavaScript, and it is not the same as an object. However, you can iterate over the keys and values of an object in a similar way using a for...in
loop or the Object.entries
method:
const obj = { key1: 'value1', key2: 'value2' };
for (const key in obj) {
console.log(key, obj[key]);
}
This will output:
key1 value1
key2 value2
You can also use the Object.entries
method to get an array of key-value pairs, which you can then iterate over using a for...of
loop:
for (const [key, value] of Object.entries(obj)) {
console.log(key, value);
}
This will have the same output as the for...in
loop above.
FAQ?
What is a map iterator in JavaScript?
In JavaScript, a map iterator is an object that allows you to iterate over the keys and values of a map. You can get a map iterator by calling the keys()
, values()
, or entries()
method of a map object.
How to iterate an array in JavaScript using a map?
You can use the map()
method to iterate over an array and perform a transformation on each element. The map()
method returns a new array with the transformed elements.
Are maps in JavaScript iterable?
Yes, maps in JavaScript are iterable, which means you can use a for...of
loop or other iteration methods (such as keys()
, values()
, and entries()
) to iterate over the keys and values of a map.
Read More.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.