When working with PHP explode Multiple Separators, one of the most common tasks developers encounter is string manipulation. Among the many string functions available in PHP, the explode()
function is widely used to split a string into an array based on a delimiter. However, things can get a bit tricky when you need to split a string using multiple delimiters or separators. Unfortunately, the native explode()
function only allows a single delimiter. In this article, we’ll explore how to handle multiple separators in PHP and split strings more effectively.
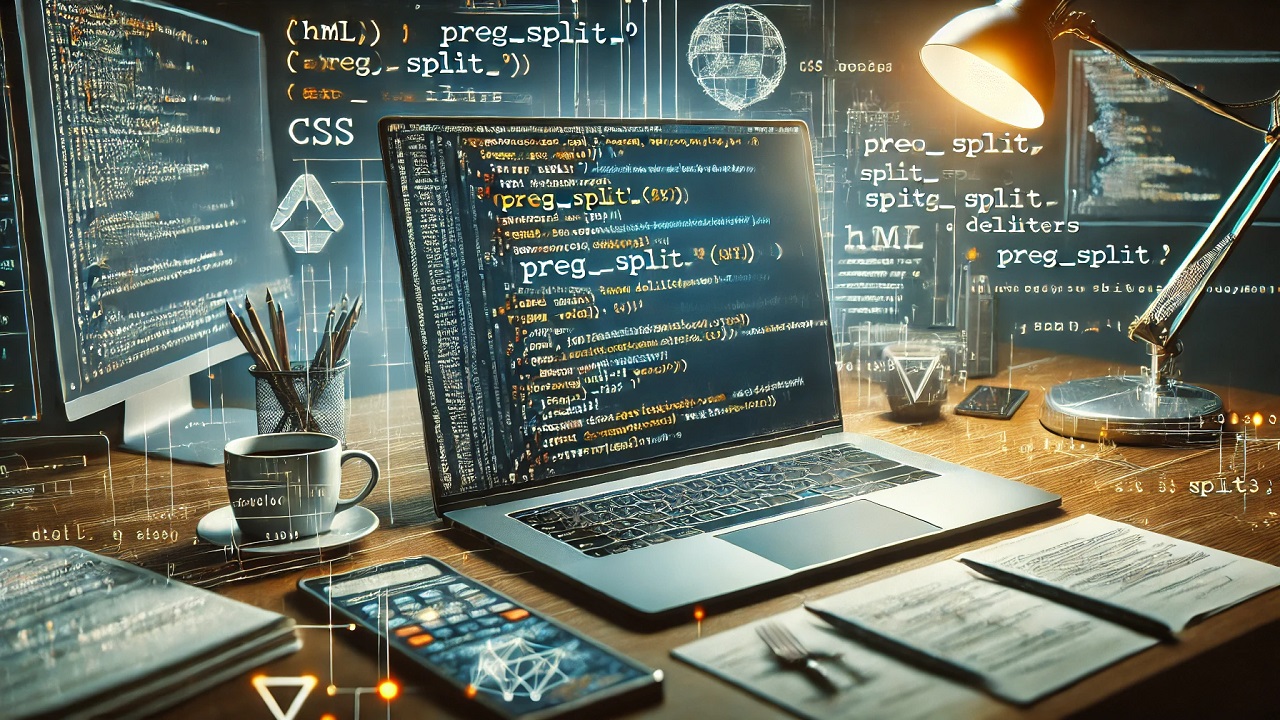
Table of Contents
PHP explode Multiple Separators List
1. Introduction to the PHP explode Multiple Separators explode()
Function
The explode()
function in PHP is a simple and effective way to break down a string into an array. Here’s the basic syntax:
$array = explode(string $delimiter, string $string [, int $limit]);
$delimiter
: The boundary string where the string will be split.$string
: The input string you want to break up.$limit
: Optional. Limits the number of splits, useful when you need a specific number of chunks.
For example, splitting a comma-separated list of fruits:
<?php
$string = "apple,banana,grape";
$result = explode(",", $string);
print_r($result);
?>
Output:
Array
(
[0] => apple
[1] => banana
[2] => grape
)
While explode()
is convenient, it only supports splitting by one delimiter. What happens when your string contains multiple delimiters?
2. Why Use Multiple Separators?
In real-world applications, data often comes in various formats, where multiple delimiters or separators are used to separate values. For example:
- User input data could have commas, pipes (
|
), and semicolons (;
) as delimiters. - Log files or external data sources may include inconsistent separators.
If you attempt to split such a string using explode()
, you’d be forced to call the function multiple times, making the code less efficient and harder to manage.
For example, consider the following string:
$string = "apple,orange|banana;grape";
The string contains three different delimiters: a comma, a pipe, and a semicolon. How can we handle this efficiently? Enter preg_split()
.
3. Using preg_split()
for Multiple Separators
PHP offers a more powerful function for splitting strings: preg_split()
. This function allows you to define a regular expression, which makes it possible to split a string using multiple delimiters.
Here’s the syntax of preg_split()
:
$array = preg_split(string $pattern, string $subject [, int $limit = -1 [, int $flags = 0]]);
$pattern
: The regular expression that defines the delimiters.$subject
: The string to split.$limit
: Optional. Limits the number of splits.$flags
: Optional. Modifies behavior, such as removing empty values or preserving delimiters.
4. Example: Splitting a String with Multiple Delimiters
Let’s go back to our earlier example string: apple,orange|banana;grape
. To split this string using preg_split()
, we can define a regular expression pattern that includes all the delimiters:
<?php
$string = "apple,orange|banana;grape";
$delimiters = "/[,|;]+/"; // Use comma, pipe, and semicolon as delimiters
$result = preg_split($delimiters, $string);
print_r($result);
?>
Output:
Array
(
[0] => apple
[1] => orange
[2] => banana
[3] => grape
)
In the above example, the regular expression /[,|;]+/
looks for any of the three delimiters—comma, pipe, or semicolon—and splits the string wherever they occur.
5. Common Use Cases for Multiple Separators
You might encounter various situations where splitting a string by multiple delimiters is necessary. Here are some practical examples:
- User Input Forms: A form that allows users to separate multiple email addresses with commas, semicolons, or spaces.
- Data Processing: External data from APIs or CSV files may contain multiple delimiters in a single string.
- Log Files: Parsing log entries where different fields are separated by various symbols.
Here’s an example of user input with multiple separators:
<?php
$emails = "[email protected], [email protected]; [email protected] | [email protected]";
$delimiters = "/[ ,;|]+/"; // Multiple separators: comma, space, semicolon, and pipe
$result = preg_split($delimiters, $emails);
print_r($result);
?>
Output:
Array
(
[0] => [email protected]
[1] => [email protected]
[2] => [email protected]
[3] => [email protected]
)
6. Handling Complex Scenarios with preg_split()
In some cases, you may want to include more advanced delimiters, such as whitespace or special characters. The preg_split()
function allows you to define complex patterns using regular expressions.
For instance, splitting on a combination of spaces, commas, pipes, and line breaks:
<?php
$string = "apple, orange | banana;grape
melon";
$delimiters = "/[\s,|;\n]+/";
$result = preg_split($delimiters, $string);
print_r($result);
?>
Output:
Array
(
[0] => apple
[1] => orange
[2] => banana
[3] => grape
[4] => melon
)
Here, we used the \s
(whitespace) and \n
(newline) symbols in the regular expression, making the split operation even more flexible.
7. Pros and Cons of preg_split()
vs explode()
While preg_split()
is a more powerful function than explode()
, it’s essential to weigh the pros and cons of using each.
Pros of preg_split()
:
- Multiple Separators: The main advantage is the ability to split strings using more than one delimiter.
- Regular Expressions: With the power of regex, you can define complex splitting rules.
- Flexibility: You can handle a wide variety of real-world string formats.
Cons of preg_split()
:
- Performance:
preg_split()
can be slower thanexplode()
because it uses regular expressions, which are more computationally intensive. - Complexity: Regular expressions can become complicated, especially for beginners.
In situations where you only need to split a string by one delimiter, explode()
remains a faster and simpler option.
FAQ?
1. What is the explode()
function in PHP?
The explode()
function in PHP is used to split a string into an array based on a single delimiter. It’s commonly used to break down strings like comma-separated values (CSV) into individual elements.
2. Can explode()
handle multiple separators?
No, the explode()
function in PHP only allows splitting by a single delimiter. To handle multiple separators, you’ll need to use preg_split()
.
3. What is the difference between explode()
and preg_split()
?
explode()
can only split a string using one specific delimiter, while preg_split()
allows splitting a string using multiple delimiters, thanks to regular expressions. This makes preg_split()
more versatile but slightly slower than explode()
.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.