To build a chat system in PHP, you will need to follow these steps:
Build a PHP project.
Set up a web server and install PHP. This can be done by installing a local server like XAMPP or by setting up a web hosting account and uploading your PHP files to it.
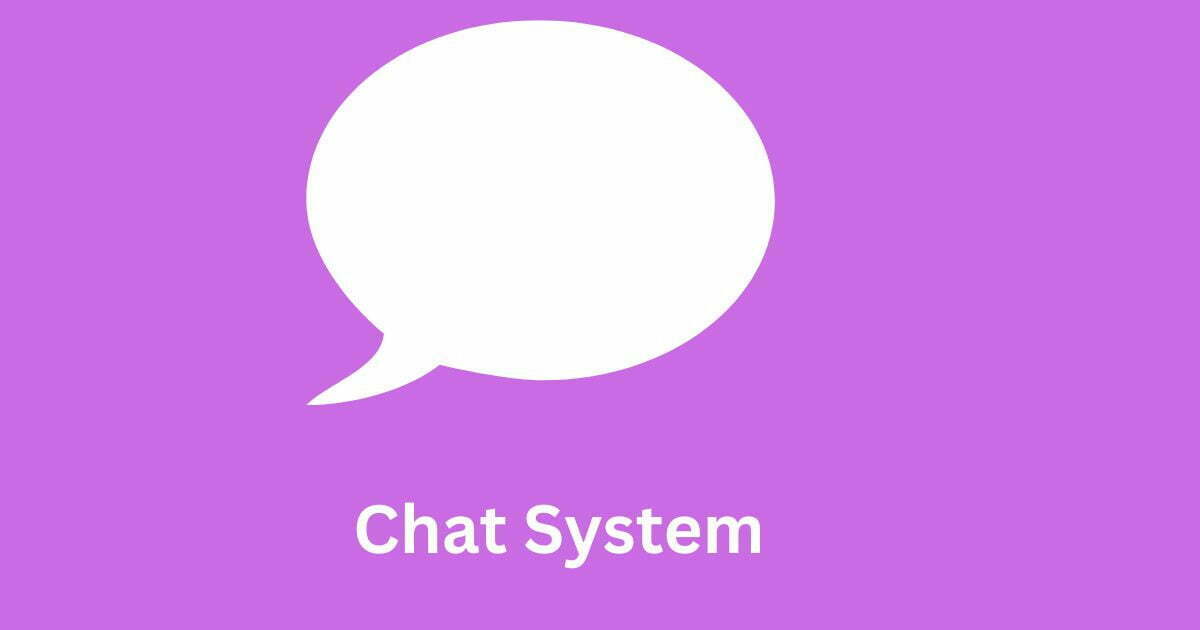
Table of Contents
Design the layout and functionality of your chat system. This will involve deciding on the features you want to include, such as the ability to send and receive messages, create and join chat rooms, and send files.
Create a database to store the chat messages and user information. You can use a database management system like MySQL or a NoSQL database like MongoDB.
Write the PHP code to handle the chat functionality. This will involve creating forms to send and receive messages and using PHP and SQL to query the database and display the messages in real-time.
Test and debug your chat system to make sure it is functioning properly.
Deploy your chat system to your web server and make it accessible to users.
Overall, building a chat system in PHP requires a combination of front-end design skills, knowledge of PHP and database management, and the ability to troubleshoot and debug issues that may arise. It can be a challenging project, but with determination and the right resources, you can create a functional and user-friendly chat system.
chat system in PHP code
Here is an example of a basic chat system in PHP:
- First, set up a database to store the chat messages. You can use a database management system like MySQL or a NoSQL database like MongoDB.
- Create a form for users to enter their messages, with a text field and a submit button.
<form action="send_message.php" method="post">
<label for="message">Message:</label><br>
<input type="text" id="message" name="message"><br>
<input type="submit" value="Send">
</form>
3. In the send_message.php
the script, use PHP to insert the message into the database and redirect the user back to the chat page.
<?php
// Connect to the database
$conn = mysqli_connect("localhost", "username", "password", "database_name");
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Escape user inputs for security
$message = mysqli_real_escape_string($conn, $_POST['message']);
// Insert message into the database
$sql = "INSERT INTO chat_messages (message) VALUES ('$message')";
if (mysqli_query($conn, $sql)) {
// Redirect the user back to the chat page
header("Location: chat.php");
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
// Close connection
mysqli_close($conn);
?>
4. On the chat page, use PHP and SQL to query the database and display the messages.
<?php
// Connect to the database
$conn = mysqli_connect("localhost", "username", "password", "database_name");
// Check connection
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
// Retrieve messages from the database
$sql = "SELECT * FROM chat_messages ORDER BY id DESC";
$result = mysqli_query($conn, $sql);
// Display the messages
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
echo $row['message'] . "<br>";
}
} else {
echo "No messages found.";
}
// Close connection
mysqli_close($conn);
?>
This is just a basic example, and you can add more features and functionality to your chat system as desired.
Read more.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.