Cookies and Session In PHP, a cookie is a small piece of data that is stored on the client’s computer when they visit a website. It is often used to store information that needs to be persisted across multiple pages or requests, such as a user’s login status or preferences.
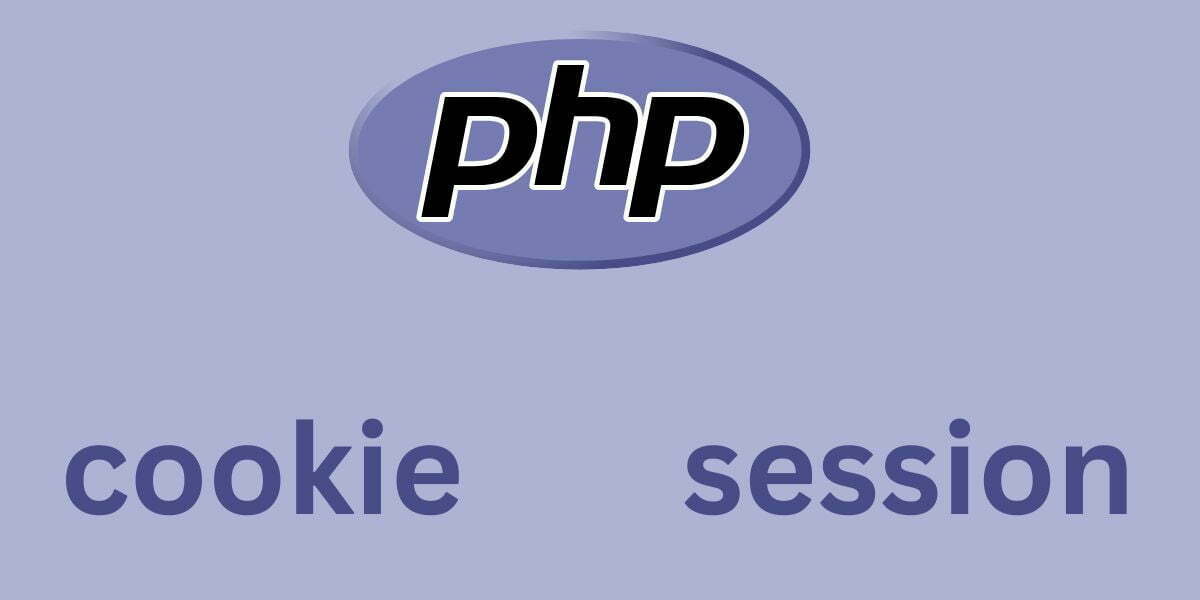
A session, on the other hand, is a way to store data on the server side that is specific to a user’s session. When a user visits a website, the server creates a new session for that user and generates a unique session ID that is sent to the client’s browser in the form of a cookie. Any data that the server wants to store and associate with the user’s session can then be stored in the session.
Table of Contents
Create Cookies and Session in PHP.
Step -1 To set a cookie in PHP, you can use the setcookie
function. For example:
setcookie('my_cookie', 'value');
Step -2 To retrieve the value of a cookie, you can use the $_COOKIE
superglobal. For example:
echo $_COOKIE['my_cookie'];
Step -1 To start a new session in PHP, you can use the session_start
function. For example:
session_start();
Step -2 To store data in the session, you can use the $_SESSION
superglobal. For example:
$_SESSION['my_session_variable'] = 'value';
Step -3 To retrieve the value of a session variable, you can use the $_SESSION
superglobal. For example:
echo $_SESSION['my_session_variable'];
Difference between cookies and session in php
Here are the main differences between cookies and sessions in PHP:
- Storage location: Cookies are stored on the client’s computer, while session data is stored on the server.
- Persistence: Cookies are persisted across multiple requests, while session data is only available during the lifetime of the session (usually until the user closes their browser).
- Size: Cookies are limited in size (usually to 4KB or less), while there is no inherent limit to the amount of data that can be stored in a session.
- Security: Cookies are stored on the client’s computer and can be accessed or modified by the client, while session data is stored on the server and is generally more secure.
Here is a summary of the main differences between cookies and sessions:
Cookies | Sessions | |
Storage location | Client’s computer | Server |
Persistence | Persisted across multiple requests | Only available during the lifetime of the session |
Size | Limited in size (usually to 4KB or less) | No inherent limit to the amount of data that can be stored |
Security | Stored on the client’s computer and can be accessed or modified by the client | Stored on the server and generally more secure |
Some Examples of cookies and session in php
Here is an example of how to create new cookies and session in PHP:
how to create cookies in PHP
To create a cookie in PHP, you can use the setcookie
function. The setcookie
the function takes at least two parameters: the name of the cookie and its value.
Here is an example of how to create a cookie in PHP:
<?php
// Set a cookie
setcookie('my_cookie', 'value');
?>
You can also specify additional optional parameters when setting a cookie.
For example, you can specify an expiration time for the cookie using the expire
parameter. If you do not specify an expiration time, the cookie will expire at the end of the current session (when the browser is closed). You can also specify a path for the cookie using the path
parameter, which determines which pages on your website will have access to the cookie.
<?php
// Set a cookie that expires in one hour
$expiration_time = time() + 3600;
setcookie('my_cookie', 'value', $expiration_time, '/');
?>
To retrieve the value of a cookie, you can use the $_COOKIE
superglobal. The $_COOKIE
superglobal is an array that contains the values of all cookies sent by the client.
For example, to retrieve the value of the my_cookie
cookie that we set above, you can do the following:
echo $_COOKIE['my_cookie'];
It’s important to note that cookies are stored on the client’s computer, which means that you have no control over what the client does with the cookie. The client can choose to disable cookies, delete cookies, or modify the value of a cookie. Therefore, it’s generally not a good idea to store sensitive information in cookies.
how to create session in PHP
To create a new session in PHP, you can use the session_start
function. This function must be called at the beginning of every page that needs to access session data.
Here is an example of how to create a new session in PHP:
<?php
// Start the session
session_start();
// Store some data in the session
$_SESSION['my_session_variable'] = 'value';
?>
The session_start function will start a new session, or resume an existing session if one already exists. It will also send a cookie to the client’s browser containing the session ID, which is used to identify the user’s session on the server.
Once a session has been started, you can store and retrieve data in the session using the $_SESSION
superglobal. The $_SESSION
superglobal is an array that can be used to store any data that you want to associate with the user’s session.
how to destroy session in php
To destroy a session in PHP, you can use the session_destroy
function. This function will destroy all data associated with the current session.
Here is an example of how to destroy a session in PHP:
<?php
// Start the session
session_start();
// Destroy the session
session_destroy();
?>
It’s important to note that the session_destroy
function only destroys the data associated with the current session, not the session itself. To start a new session, you will need to call the session_start
function again.
Alternatively, you can unset all variables in the session by unsetting the $_SESSION
superglobal:
<?php
// Start the session
session_start();
// Unset all session variables
$_SESSION = array();
?>
This will remove all data from the session, but the session itself will still exist. To completely destroy the session and the session cookie, you can use the session_unset
and session_destroy
functions in combination with the session_regenerate_id
function, like this:
<?php
// Start the session
session_start();
// Unset all session variables
session_unset();
// Destroy the session
session_destroy();
// Regenerate the session ID
session_regenerate_id(true);
?>
This will unset all session variables, destroy the session, and regenerate the session ID, effectively starting a new session.
FAQ?
in PHP where session variable values are stored MCQ
The session data is stored in a file on the server, or in a database on the server, depending on how PHP is configured. By default, PHP stores session data in files on the server, but you can also configure it to use a database, such as MySQL, to store session data. The session data is stored in a file on the server, or in a database on the server, depending on how PHP is configured. By default, PHP stores session data in files on the server, but you can also configure it to use a database, such as MySQL, to store session data.
How to create session in PHP
To create a new session in PHP, you can use the session_start
function. This function must be called at the beginning of every page that needs to access session data.
How to destroy session in php
To destroy a session in PHP, you can use the session_destroy
function. This function will destroy all data associated with the current session.
Read more Latest Post.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.