Here is a basic example of a login system using PHP and HTML:
This code first displays an HTML form that asks the user for a username and password. When the form is submitted, the PHP code runs and checks the provided username and password against a database of users.
If the login is successful, it sets a session variable to indicate that the user is logged in and redirects to the home page. If the login is unsuccessful, it displays an error message.
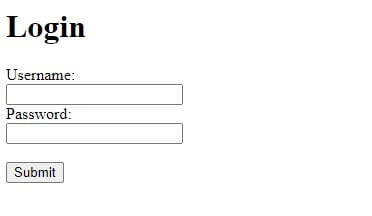
Table of Contents
login system using PHP and HTML Example:
HTML FORM:
<form action="login.php" method="post">
<label for="username">Username:</label><br>
<input type="text" id="username" name="username"><br>
<label for="password">Password:</label><br>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Submit">
</form>
PHP FILE:
<?php
// Check if the form was submitted
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
// Get the username and password from the form
$username = $_POST['username'];
$password = $_POST['password'];
// Connect to the database
$conn = new mysqli('localhost', 'dbuser', 'dbpass', 'dbname');
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Check if the username and password are correct
$result = $conn->query("SELECT * FROM users WHERE username='$username' AND password='$password'");
if ($result->num_rows > 0) {
// Set a session variable to indicate that the user is logged in
$_SESSION['logged_in'] = true;
// Redirect to the home page
header('Location: index.php');
exit;
} else {
// Display an error message
echo 'Invalid username or password';
}
}
?>
This is just a basic example and you may want to consider adding additional security measures such as hashing the passwords, using prepared statements to prevent SQL injection attacks, and implementing rate limiting to prevent brute force attacks.
Read More.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.