PHP for loop are one of the most important constructs used in programming, and they are essential when it comes to working with arrays. In this article, we will explore how to use PHP for loops with arrays to create efficient and dynamic applications.
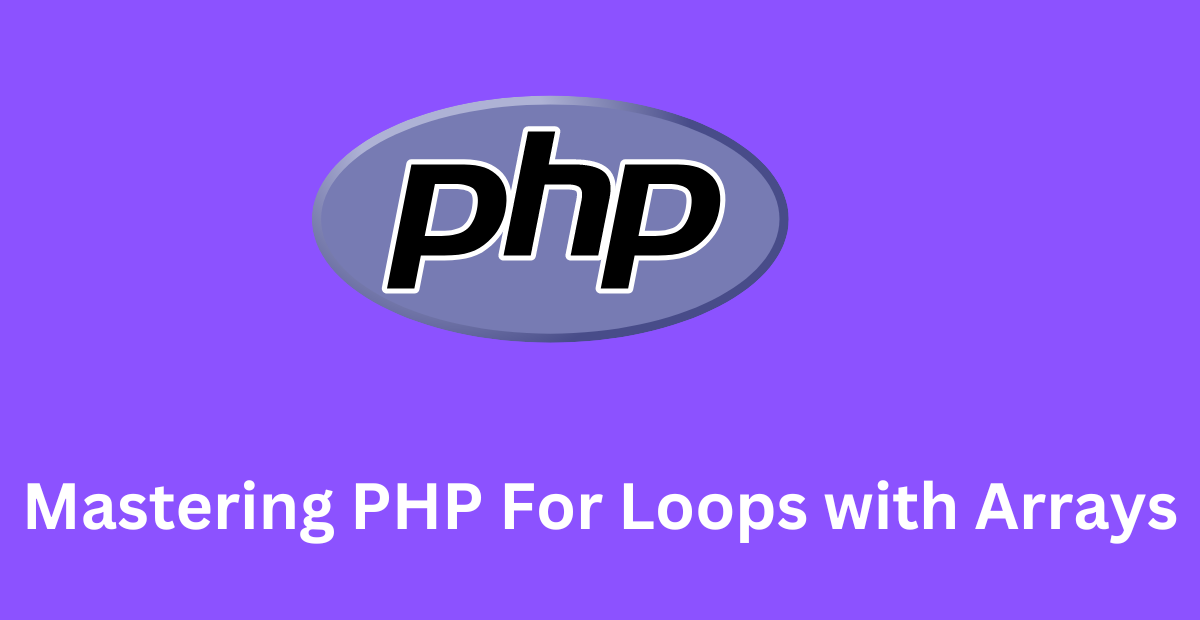
First, let’s briefly go over what arrays are and how they work in PHP.
How to use PHP for loop with Arrays to create efficient and dynamic applications
Arrays in PHP
In PHP, an array is a variable that can hold multiple values. These values can be of different data types, including strings, numbers, or even other arrays. The values in an array are referred to as elements, and each element is associated with an index number.
Here’s an example of how to declare an array in PHP:
$fruits = array("Apple", "Banana", "Orange");
This creates an array named $fruits with three elements: “Apple”, “Banana”, and “Orange”. The index numbers for these elements are 0, 1, and 2, respectively.
Using a Php for loop with an array
Now that we have an understanding of arrays in PHP, let’s explore how to use a for loop with an array.
You can use a for loop to run a block of code a certain number of times. The fundamental structure of a for loop is as follows:
for (initialization; condition; increment/decrement) {
// code to be executed
}
Here’s an example of how to use a for loop with an array to print out each element of the $fruits array we created earlier:
$fruits = array("Apple", "Banana", "Orange");
for ($i = 0; $i < count($fruits); $i++) {
echo $fruits[$i] . "<br>";
}
In this example, we first declare the $fruits array with three elements. Then, we use a for loop to iterate over each element in the array.
The count() function is used to get the total number of elements in the array. We then use the $i variable as an index to access each element of the array, starting from 0 and incrementing by 1 each time.
Inside the loop, we use the echo statement to output each element of the array, followed by a tag to create a new line after each element.
Conclusion
Using a for loop with an array is a powerful technique that allows you to iterate over each element of an array and perform specific actions on each element. In this article, we have explored how to use a for loop with an array in PHP.
By understanding the basics of arrays and how to use a for loop with them, you can create more efficient and dynamic PHP applications.
FAQ?
What is the difference between a for loop and a foreach loop in PHP?
A for loop is used to execute a block of code for a specific number of times, whereas a foreach loop is used to iterate over each element in an array or an object.
Can I use a for loop to loop through a multidimensional array in PHP?
Yes, you can use a nested for loop to loop through a multidimensional array in PHP. Here’s an example:
$products = array(
array(“Product A”, 10),
array(“Product B”, 20),
array(“Product C”, 30)
);
for ($i = 0; $i < count($products); $i++) { for ($j = 0; $j < count($products[$i]); $j++) { echo $products[$i][$j] . ” “; } echo “
“;
}
This will loop through each element of the $products
array, which is a multidimensional array, and output each element of the inner array followed by a new line after each array.
How do I remove an element from an array using a for loop in PHP?
To remove an element from an array using a for loop in PHP, you can use the unset()
function to remove a specific element based on its index. For example:
$fruits = array(“Apple”, “Banana”, “Orange”);
for ($i = 0; $i < count($fruits); $i++) {
if ($fruits[$i] == “Banana”) {
unset($fruits[$i]);
}
}
This will remove the element “Banana” from the $fruits
array.
Read more.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.