Python is a popular programming language that is widely used for a variety of tasks, including data analysis, machine learning, and web development.
One of the key features of Python Loop Through Dictionary is its ability to work with data structures such as lists, tuples, and dictionaries. In this article, we will focus on dictionaries and how to loop through them in Python.
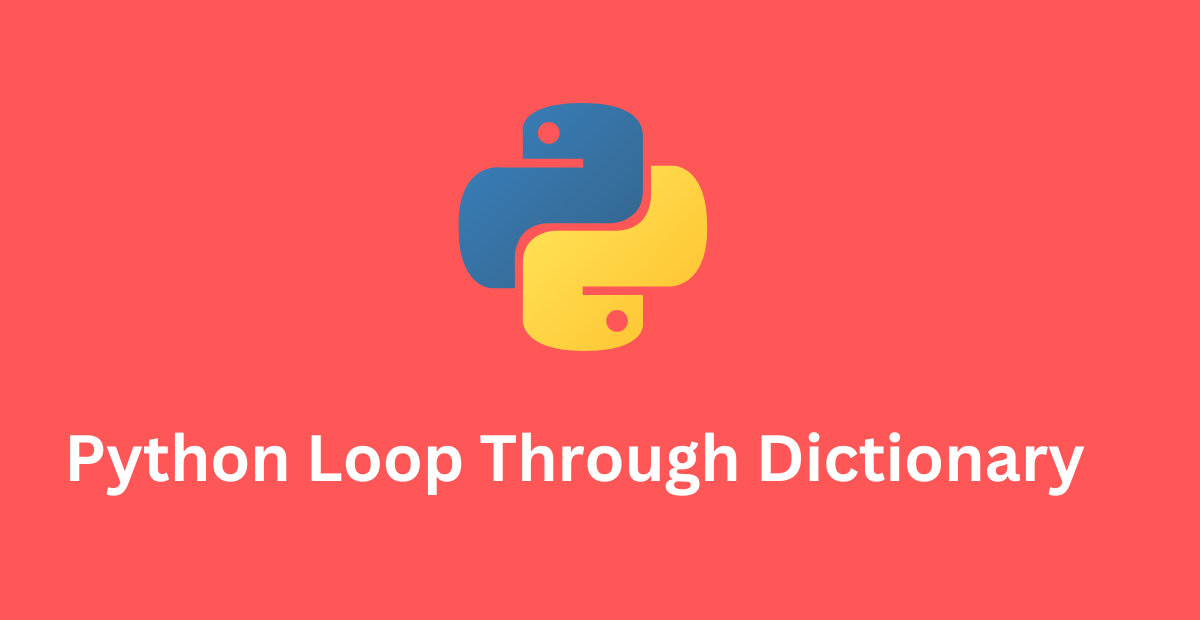
Python Loop Through Dictionary: Understanding Dictionaries in Python
Before we dive into the details of how to loop through a dictionary in Python, let’s first understand what a dictionary is and how it works.
Dictionaries are data structure that stores a collection of key-value pairs. Each key within a dictionary is associated with a specific value.
It’s important to note that dictionaries are unordered, meaning that the order of the keys within a dictionary is not fixed. Furthermore, it’s required that each key within a dictionary must be unique, and cannot be duplicated.
This is a sample representation of a Python Loop Through dictionary:
my_dict = {"apple": 1, "banana": 2, "orange": 3}
In this dictionary, the keys are “apple”, “banana”, and “orange”, and the corresponding values are 1, 2, and 3, respectively.
To access a value in a dictionary, you can use the key as follows:
my_dict["apple"] # returns 1
You can also add new key-value pairs to a dictionary using the following syntax:
my_dict["grape"] = 4
This adds a new key-value pair to the dictionary where the key is “grape” and the value is 4.
Python Loop Through Dictionary: Looping Through a Dictionary in Python
Now that we have a basic understanding of dictionaries in Python, let’s move on to how to loop through a dictionary.
There are several ways to loop through a dictionary in Python, but the most common way is to use a for loop. Here’s an example:
my_dict = {"apple": 1, "banana": 2, "orange": 3}
for key in my_dict:
value = my_dict[key]
print(key, value)
This code will output the following:
apple 1
banana 2
orange 3
In this for loop, we first iterate over the keys in the dictionary using the syntax for key in my_dict
. Then, for each key, we retrieve the corresponding value using the syntax value = my_dict[key]
. Finally, we print both the key and the value using the print
statement.
You can also loop through just the keys or just the values of a dictionary using the keys()
and values()
methods, respectively. Here’s an example:
my_dict = {"apple": 1, "banana": 2, "orange": 3}
# Loop through just the keys
for key in my_dict.keys():
print(key)
# Loop through just the values
for value in my_dict.values():
print(value)
This code will output the following:
apple
banana
orange
1
2
3
Practical Applications of Python Loop Through Dictionary.
Looping through a dictionary is a common task in Python programming, and it has many practical applications. Here are a few examples:
- Data Analysis: If you have a dictionary of data that you want to analyze, you can use a loop to iterate over the keys and values in the dictionary and perform various calculations or analyses on the data. For example, you could use a loop to calculate the average value of a set of data or to identify the maximum and minimum values in a dataset.
- Web Development: Dictionaries are commonly used in web development to store data such as user information, product details, and website settings. By looping through a dictionary, you can easily access and manipulate this data in your web application.
- Machine Learning: Machine learning algorithms often use dictionaries to represent data or parameters. By looping through a dictionary, you can easily retrieve and update these parameters during the training process.
Python provides a versatile approach to looping through dictionaries, which can be leveraged for various use cases. Whether you’re working on a data analysis project, a web application, or a machine learning algorithm, proficiently iterating through a dictionary can enhance your productivity and efficiency while working.
Conclusion:
In conclusion, dictionaries are a powerful data structure in Python that allows you to store and manipulate key-value pairs. By looping through a dictionary, you can easily access and manipulate the data stored within it. Whether you are working on a data analysis project, a web application, or a machine learning algorithm, being able to efficiently loop through a dictionary will help you to be more productive and effective in your work.
User Solve Query.
#python iterate dictionary key, value
#python loop through dictionary of dataframes
#python loop through list
#python iterate through dictionary with multiple values
#how to store values in dictionary in python using for loop
Read more.