A python class static method in Python is a method that is an instance of a class rather than a member of it. It can be accessed using the class name rather than an instance of the class.
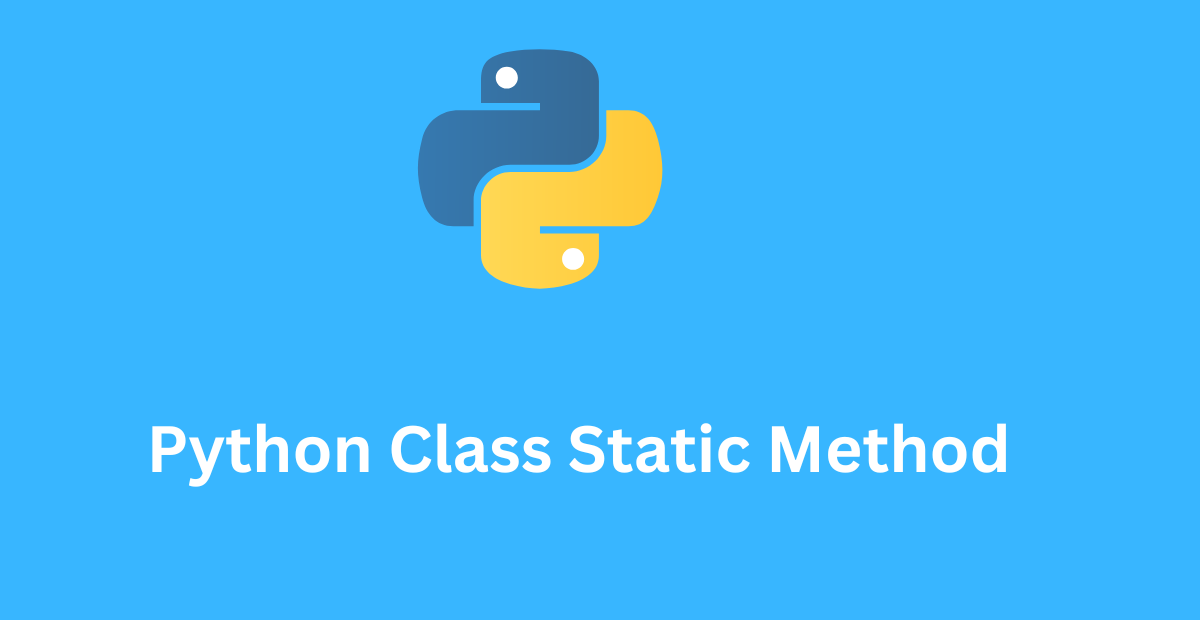
Following Example of the Python Class Static Method.
To define a static method in a Python class, you need to use the @staticmethod decorator before the method definition. Here’s an example:
class MyClass:
@staticmethod
def my_static_method(arg1, arg2):
# method code goes here
In the above example, my_static_method is a static method in the MyClass class. It can be called using the class name, like this:
MyClass.my_static_method(arg1_value, arg2_value)
Note:- that static methods don’t have access to the instance of the class or its attributes. They’re mostly used for utility functions that don’t require any class or instance-level information.
Static methods are useful when you need to define a method that’s related to the class but doesn’t rely on any instance-level information. They can also help improve the performance of your code by avoiding the overhead of creating an instance of the class.
Read More.
- The Ultimate Guide to the Top 10 Java Frameworks for 2024.
- A Comprehensive Guide to Using javascript:location.reload(true) in Web Development
- PHP explode Multiple Separators: A Comprehensive Guide.
- Copy Constructor in Java: A Complete Guide
- 50 Ultimate PHP Project Topics to Elevate Your Development Skills.