Java, a versatile and widely used programming language, provides various data structures to facilitate efficient data manipulation. Among these, the ArrayList stands out as a dynamic and resizable array implementation that brings flexibility to handling collections of elements.
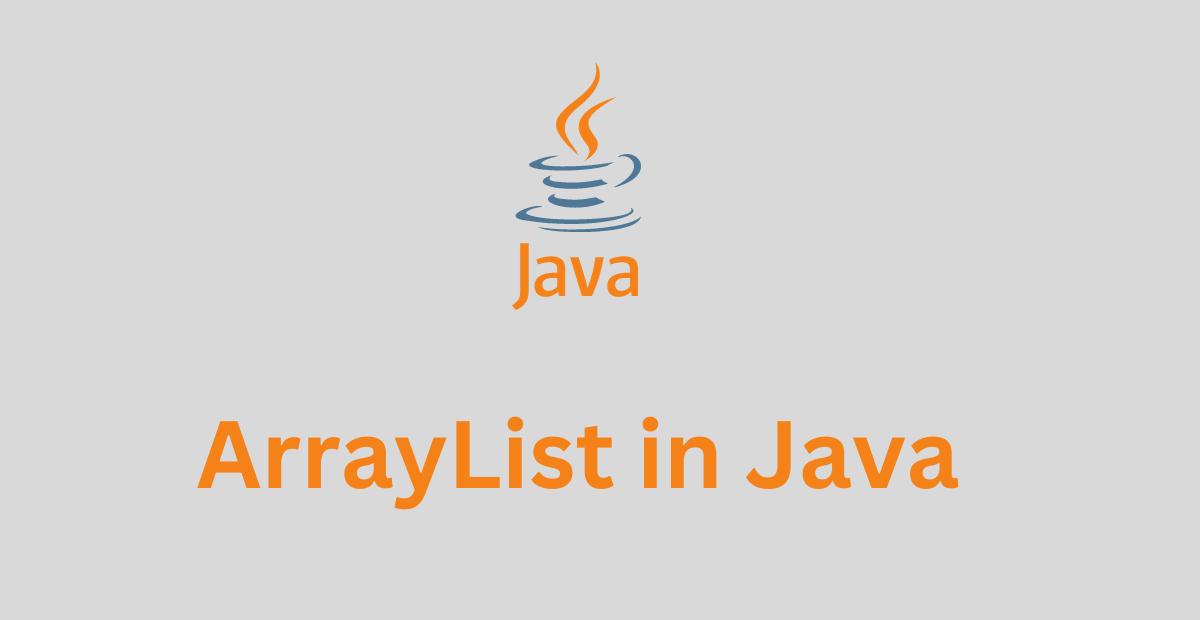
In this article, we’ll delve into the intricacies of ArrayList in Java, exploring its features, advantages, and best practices for optimal utilization.
Introduction to ArrayList in Java
At its core, an ArrayList is a part of the Java Collections Framework and belongs to the java.util package. Unlike traditional arrays, ArrayLists can dynamically grow or shrink in size, making them particularly advantageous when dealing with situations where the number of elements is unknown or subject to change.
Dynamic Sizing
One of the key features of ArrayList is its dynamic sizing capability. Unlike static arrays, where the size is fixed, ArrayLists can resize themselves automatically when elements are added or removed. This dynamic behavior simplifies coding and eliminates the need for manual resizing operations.
Declaration and Initialization
To declare and initialize an ArrayList in Java, you use the ArrayList class. For instance:
ArrayList<String> myArrayList = new ArrayList<>();
This line creates an ArrayList capable of holding strings. The angle brackets (<>
) denote generics, allowing type-safe collections.
Adding and Removing Elements
ArrayList provides methods like add()
and remove()
to insert and delete elements, respectively. These operations are executed efficiently, with the underlying resizing managed seamlessly by the ArrayList class.
myArrayList.add("Java");
myArrayList.add("ArrayList");
myArrayList.remove("Java");
Iterating Through ArrayList
Traversal of elements is a common operation, and ArrayList simplifies it with enhanced for loops or iterators.
for (String element : myArrayList) {
System.out.println(element);
}
Advantages of ArrayList
- Dynamic Resizing: As mentioned earlier, the ability to resize dynamically makes ArrayLists adaptable to varying data sizes, ensuring efficient memory usage.
- Built-in Methods: ArrayList comes with numerous built-in methods for common operations like sorting, searching, and more. This reduces the need for developers to implement these functionalities from scratch.
- Type Safety: Generics in ArrayLists enforce type safety, preventing the inadvertent introduction of incompatible types into the collection.
Best Practices
1.Specify Initial Capacity: If you have an estimate of the number of elements, providing an initial capacity when creating an ArrayList can enhance performance by reducing the number of resizing operations.
ArrayList<String> myArrayList = new ArrayList<>(20);
- Use Enhanced For Loop: When iterating through elements, favor the enhanced for loop or iterators for concise and readable code.
- Consider ArrayList Alternatives: Depending on specific use cases, alternatives like LinkedList or HashSet may offer better performance. Evaluate your requirements to choose the most suitable collection type.
Conclusion
In conclusion, the ArrayList in Java stands as a powerful tool for managing dynamic collections of data. Its dynamic sizing, built-in methods, and type safety contribute to its widespread use in various applications. By understanding its features and adhering to best practices, developers can harness the full potential of ArrayLists in Java, enhancing the efficiency and maintainability of their code.
FAQ?
What is an ArrayList in Java?
An ArrayList in Java is a dynamic array implementation that belongs to the Java Collections Framework. It provides dynamic resizing, allowing developers to create resizable arrays to store elements of any data type.
How is an ArrayList different from a regular array in Java?
Unlike regular arrays in Java, ArrayLists can dynamically grow or shrink in size. They provide methods for easy insertion, deletion, and traversal of elements, eliminating the need for manual resizing and making them more flexible.
What are the advantages of using ArrayList?
Some advantages of ArrayList include dynamic resizing, built-in methods for common operations, type safety through generics, and ease of use in managing collections of varying sizes.
Are there any best practices for using ArrayList?
Best practices include specifying an initial capacity if possible, using enhanced for loops for iteration, and considering alternative collection types based on specific use cases.
Read More